Building a Robust Web Application with Flask and React: A Comprehensive Guide
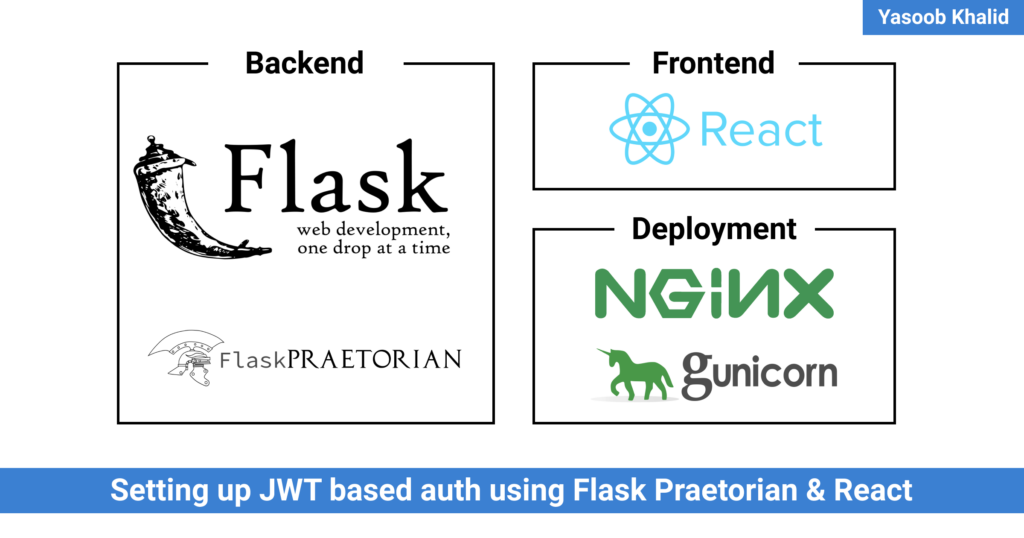
Introduction
This in-depth guide will walk you through the process of constructing a full-fledged web application using the powerful combination of Flask and React. We’ll delve into the fundamentals of both frameworks, their integration, and best practices for building scalable and maintainable applications.
Understanding Flask and React
Flask: The Pythonic Microframework
Flask, a Python-based microframework, provides the foundation for building web applications with remarkable flexibility and efficiency. Its minimalist approach empowers developers to create custom solutions tailored to specific project requirements.
Core Features:
- Routing: Define URL patterns and corresponding functions.
- Request Handling: Process incoming requests and generate responses.
- Templating: Create dynamic HTML pages using Jinja2.
- Error Handling: Manage exceptions gracefully.
Key Benefits:
- Simplicity: Easy to learn and use.
- Flexibility: Highly customizable.
- Performance: Efficient for small to medium-sized applications.
- Large Ecosystem: Extensive community and third-party libraries.
React: Building User Interfaces
React, a JavaScript library developed by Facebook, excels at crafting interactive and dynamic user interfaces. Its component-based architecture promotes code reusability and maintainability.
Core Concepts:
- Components: Reusable building blocks of UI.
- JSX: Syntax extension for embedding HTML-like structures in JavaScript.
- Virtual DOM: Efficiently updates the UI.
- State and Props: Manage component data and properties.
Key Benefits:
- Declarative Style: Describe the desired UI state.
- Component-Based Architecture: Modular and scalable.
- Performance Optimization: Virtual DOM for efficient updates.
- Large Community: Extensive support and resources.
Setting Up the Development Environment
Before diving into the code, ensure your development environment is configured correctly.
- Install Python and Node.js: Download and install the latest versions of Python and Node.js.
- Create a Virtual Environment: Isolate project dependencies using
virtualenv
orvenv
. - Install Flask and React: Use
pip
andnpm
(oryarn
) to install Flask, React, and other required packages.
Building the Flask Backend
Project Structure
Create a well-organized project structure to separate different components:
project_name/
├── app/
│ ├── __init__.py
│ ├── models.py
│ ├── routes.py
│ └── utils.py
├── config.py
├── requirements.txt
├── run.py
└── tests/
Database Integration (Optional)
If your application requires data persistence, consider using a database like SQLAlchemy:
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy()
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
API Endpoints
Define API endpoints to expose data and functionality to the frontend:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/users', methods=['GET'])
def get_users():
users = [
{'id': 1, 'name': 'John Doe'},
{'id': 2, 'name': 'Jane Smith'}
]
return jsonify(users)
Error Handling
Implement proper error handling to provide informative messages to the user:
from flask import jsonify
@app.errorhandler(404)
def not_found(error):
return jsonify({'error': 'Not found'}), 404
Creating the React Frontend
Project Structure
Organize your React project with clear directory conventions:
frontend/
├── public/
│ ├── index.html
│ └── ...
├── src/
│ ├── App.js
│ ├── components/
│ │ ├── Header.js
│ │ ├── Footer.js
│ │ └── ...
│ ├── services/
│ │ └── api.js
│ ├── styles/
│ │ └── App.css
│ └── ...
Component Structure
Break down the UI into reusable components for better organization and maintainability:
import React from 'react';
function Header() {
return (
<header>
<h1>My Application</h1>
</header>
);
}
export default Header;
State Management (Optional)
For complex applications, consider using state management libraries like Redux or Zustand:
import { createStore } from 'redux';
const initialState = {
users: []
};
function reducer(state = initialState, action) {
switch (action.type) {
case 'FETCH_USERS_SUCCESS':
return { ...state, users: action.payload };
default:
return state;
}
}
const store = createStore(reducer);
API Interactions
Fetch data from the Flask backend using fetch
or libraries like axios
:
import axios from 'axios';
async function fetchUsers() {
const response = await axios.get('/api/users');
return response.data;
}
Integrating Flask and React
Development Server
Run both Flask and React development servers simultaneously:
# In a separate terminal for Flask
flask run
# In another terminal for React
npm start
Proxy Configuration (Optional)
If using a different port for the backend, configure a proxy in React to simplify API calls:
// package.json
"proxy": "http://localhost:5000"
Deployment
Deploy your application to a production environment using platforms like Heroku, AWS, or Google Cloud:
- Backend Deployment: Package your Flask application as a Python package.
- Frontend Deployment: Build your React application for production and deploy static files.
Additional Considerations
- Security: Implement robust security measures to protect user data.
- Performance Optimization: Optimize your application for speed and responsiveness.
- Testing: Write comprehensive unit and integration tests.
- Code Quality: Adhere to coding standards and style guides.
- Deployment Automation: Automate the deployment process for efficiency.
Conclusion
By following this comprehensive guide, you’ve gained a solid foundation for building web applications with Flask and React. Experiment with different features, libraries, and design patterns to create innovative and engaging user experiences.