Developing LabVIEW Applications for Robotics
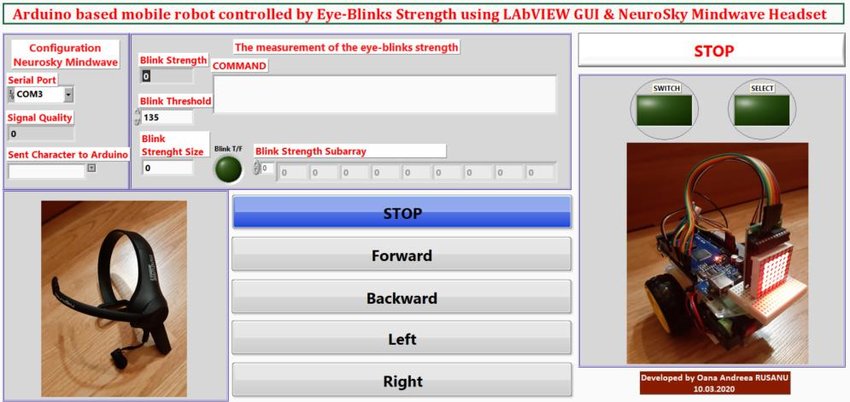
Introduction
LabVIEW (Laboratory Virtual Instrument Engineering Workbench) is a powerful graphical programming environment widely used in engineering and scientific applications. Its intuitive interface and vast library of functions make it an ideal tool for developing robotics applications. Robotics, a multidisciplinary field encompassing mechanical engineering, electrical engineering, computer science, and control theory, benefits greatly from the versatility and capabilities of LabVIEW.
This article provides an in-depth exploration of developing LabVIEW applications for robotics. We will cover the fundamentals of LabVIEW, the architecture of robotic systems, integration of sensors and actuators, control algorithms, simulation, and real-world deployment. By the end of this article, you should have a comprehensive understanding of how to leverage LabVIEW for developing robust and efficient robotic systems.
Fundamentals of LabVIEW
Overview of LabVIEW
LabVIEW, developed by National Instruments, is a system-design platform and development environment focused on data acquisition, instrument control, and industrial automation. Unlike traditional text-based programming languages, LabVIEW utilizes a graphical programming approach, where developers create programs by connecting functional blocks with wires in a visual diagram.
Key Features
- Graphical Programming Interface: LabVIEW’s visual programming language, G, allows developers to create programs using block diagrams. This makes it easier to visualize the flow of data and control logic.
- Comprehensive Libraries: LabVIEW offers extensive libraries for data acquisition, signal processing, control design, and communication protocols.
- Hardware Integration: LabVIEW supports a wide range of hardware devices, including sensors, actuators, data acquisition cards, and embedded systems.
- Real-Time and FPGA Capabilities: LabVIEW’s real-time and FPGA modules enable the development of high-performance applications with precise timing requirements.
- Cross-Platform Development: LabVIEW applications can be deployed on various platforms, including Windows, macOS, Linux, and real-time operating systems.
LabVIEW Development Environment
The LabVIEW development environment consists of several key components:
- Front Panel: The graphical user interface (GUI) where users interact with the application. It includes controls (inputs) and indicators (outputs) for displaying data.
- Block Diagram: The workspace for creating the program logic. It consists of nodes (functions, structures) and wires (data paths) that define the data flow.
- Palette: A collection of functions and structures organized into categories, such as Mathematics, Signal Processing, and Instrument I/O.
- Tools Palette: A set of tools for editing the front panel and block diagram, such as the wiring tool, text tool, and probe tool.
- Context Help: Provides detailed information about the functions and structures used in the block diagram.
Basic Programming Concepts
- Data Flow: LabVIEW programs, or VIs (Virtual Instruments), are based on the principle of data flow. Execution is determined by the flow of data between nodes, rather than a predefined sequence of instructions.
- Structures: Control the flow of execution and data. Common structures include loops (For Loop, While Loop), conditionals (Case Structure), and sequences (Flat Sequence, Stacked Sequence).
- SubVIs: Reusable VIs that encapsulate specific functionality. SubVIs help organize and modularize code, making it easier to maintain and debug.
- Data Types: LabVIEW supports various data types, including numeric, boolean, string, array, and cluster. Proper use of data types ensures efficient data handling and processing.
Architecture of Robotic Systems
Components of a Robotic System
Robotic systems typically consist of several key components:
- Sensors: Devices that measure physical quantities such as distance, temperature, pressure, and light. Common sensors used in robotics include ultrasonic sensors, infrared sensors, cameras, and accelerometers.
- Actuators: Devices that convert electrical signals into mechanical motion. Examples include motors, servos, and pneumatic actuators.
- Controllers: The brain of the robot, responsible for processing sensor data and generating control signals for the actuators. Controllers can be microcontrollers, PLCs, or embedded computers.
- Power Supply: Provides the necessary electrical power to the sensors, actuators, and controllers.
- Communication Interfaces: Enable data exchange between the robot and external devices or systems. Common interfaces include UART, I2C, SPI, CAN, and Ethernet.
System Integration
Integrating the various components of a robotic system involves:
- Interfacing Sensors and Actuators: Connecting sensors and actuators to the controller and configuring their operation.
- Signal Conditioning: Processing sensor signals to ensure they are suitable for use by the controller. This may involve amplification, filtering, and analog-to-digital conversion.
- Control Algorithms: Implementing algorithms to process sensor data and generate appropriate control signals for the actuators. Common algorithms include PID control, state machines, and kinematic models.
- Communication Protocols: Establishing communication between the robot and external devices or systems using standard protocols.
Design Considerations
When designing a robotic system, several factors must be considered:
- System Requirements: Define the functional and performance requirements of the robot, including speed, accuracy, payload, and operating environment.
- Modularity: Design the system with modular components to facilitate maintenance, upgrades, and scalability.
- Reliability: Ensure the system is robust and reliable, with appropriate fault detection and recovery mechanisms.
- Safety: Incorporate safety features to protect both the robot and its operators from harm.
- Cost: Optimize the system design to balance performance and cost.
Developing LabVIEW Applications for Robotics
Setting Up the Development Environment
- Install LabVIEW: Obtain and install LabVIEW from National Instruments’ website. Ensure you have the necessary modules for your application, such as the Real-Time and FPGA modules.
- Hardware Setup: Connect your sensors, actuators, and controllers to your development system. Ensure all drivers and firmware are up to date.
- Project Organization: Create a new LabVIEW project and organize your VIs, libraries, and hardware configurations.
Interfacing with Sensors and Actuators
- Sensor Integration:
- Digital Sensors: Connect digital sensors using GPIO (General Purpose Input/Output) or communication interfaces like I2C, SPI, or UART. Use LabVIEW’s built-in functions to read sensor data.
- Analog Sensors: Connect analog sensors to analog input channels. Use signal conditioning techniques to ensure accurate measurements. Utilize LabVIEW’s data acquisition functions to read analog signals.
- Actuator Control:
- Motors and Servos: Use LabVIEW’s motor control functions to generate PWM (Pulse Width Modulation) signals for controlling motors and servos. Implement feedback control loops to achieve precise motion control.
- Pneumatic Actuators: Interface with pneumatic actuators using solenoid valves and digital outputs. Implement control algorithms to manage the operation of pneumatic systems.
Implementing Control Algorithms
- PID Control:
- Proportional-Integral-Derivative (PID) Control: Implement PID controllers to regulate the behavior of the robot. Use LabVIEW’s PID toolkit to design and tune PID controllers.
- Tuning PID Parameters: Adjust the proportional, integral, and derivative gains to achieve desired performance. Use techniques like Ziegler-Nichols or trial-and-error for tuning.
- State Machines:
- Finite State Machines (FSM): Implement FSMs to manage the different operating modes and transitions of the robot. Use LabVIEW’s state machine templates to create and manage states and transitions.
- Event-Driven Programming: Use event structures to handle asynchronous events and triggers in the robot’s operation.
- Kinematic Models:
- Forward and Inverse Kinematics: Implement kinematic models to calculate the position and orientation of the robot’s end-effector. Use LabVIEW’s mathematical functions to solve kinematic equations.
- Trajectory Planning: Develop algorithms to plan smooth and collision-free trajectories for the robot. Use techniques like cubic splines and polynomial interpolation.
Data Acquisition and Processing
- Data Acquisition:
- Real-Time Data Acquisition: Use LabVIEW’s data acquisition functions to capture real-time data from sensors. Ensure proper timing and synchronization to avoid data loss.
- Buffered Acquisition: Implement buffered data acquisition to handle high-speed data streams and avoid overloading the processor.
- Data Processing:
- Signal Filtering: Apply filters to remove noise and unwanted frequencies from sensor signals. Use LabVIEW’s signal processing toolkit for designing and implementing filters.
- Data Analysis: Perform data analysis to extract meaningful information from sensor data. Use techniques like FFT (Fast Fourier Transform), statistical analysis, and pattern recognition.
Simulation and Testing
- Simulation:
- Virtual Prototyping: Create virtual prototypes of the robot using LabVIEW’s simulation toolkit. Simulate the behavior of the robot to validate design concepts and algorithms.
- Hardware-in-the-Loop (HIL) Simulation: Combine simulation with real hardware components to test the interaction between the virtual and physical systems.
- Testing:
- Unit Testing: Test individual components and modules to ensure they function correctly. Use LabVIEW’s testing tools to automate and document tests.
- Integration Testing: Test the integrated system to verify that all components work together as expected. Identify and resolve any issues that arise during integration.
- Performance Testing: Evaluate the performance of the robot under different operating conditions. Measure parameters like speed, accuracy, and power consumption.
Deployment and Maintenance
- Deploying Applications:
- Real-Time Targets: Deploy LabVIEW applications to real-time targets like CompactRIO or PXI systems. Ensure real-time performance and deterministic behavior.
- Embedded Systems: Deploy applications to embedded systems with limited resources. Optimize code to fit within the constraints of the target platform.
- Maintenance:
- Monitoring and Diagnostics: Implement monitoring and diagnostic tools to detect and troubleshoot issues in the robot’s operation. Use LabVIEW’s remote monitoring capabilities to access the robot from a remote location.
- Software Updates: Develop procedures for updating the robot’s software to incorporate new features and improvements. Ensure updates do not disrupt the robot’s operation.
- Documentation: Maintain comprehensive documentation of the robot’s design, implementation, and operation. Use LabVIEW’s documentation tools to generate and organize documentation.
Case Studies
Case Study 1: Autonomous Mobile Robot
Project Overview: Develop an autonomous mobile robot for warehouse automation. The robot should navigate through the warehouse, avoid obstacles, and transport goods between locations.
- System Design:
- Sensors: Use LIDAR and ultrasonic sensors for obstacle detection and avoidance. Use encoders for measuring wheel rotations and calculating position.
- Actuators: Use DC motors with encoders for driving the wheels. Implement motor control using PWM signals.
- Controller: Use a CompactRIO system with a real-time controller for processing sensor data and controlling the motors.
- Algorithm Development:
- Navigation: Implement a navigation algorithm using the A* search algorithm for path planning. Use LabVIEW’s mathematical functions to calculate optimal paths.
- Obstacle Avoidance: Implement an obstacle avoidance algorithm using sensor data. Use LabVIEW’s PID toolkit to control the robot’s speed and direction.
- Localization: Use encoders and LIDAR data to implement a localization algorithm based on the Kalman filter.
- Simulation and Testing:
- Virtual Simulation: Create a virtual model of the warehouse and simulate the robot’s navigation and obstacle avoidance. Validate the algorithm’s performance in the simulation environment.
- Field Testing: Test the robot in a real warehouse environment. Collect data to identify and resolve any issues in the robot’s operation.
- Deployment:
- Real-Time Deployment: Deploy the application to the CompactRIO system. Ensure real-time performance and robustness.
- Monitoring: Implement remote monitoring to track the robot’s operation and performance. Use LabVIEW’s web services for remote access.
Case Study 2: Robotic Arm for Precision Assembly
Project Overview: Develop a robotic arm for precision assembly tasks in a manufacturing environment. The robotic arm should accurately position and assemble components with high repeatability.
- System Design:
- Sensors: Use vision sensors for detecting and positioning components. Use force/torque sensors for monitoring assembly forces.
- Actuators: Use servo motors with encoders for controlling the joints of the robotic arm. Implement closed-loop control for precise positioning.
- Controller: Use a PXI system with an embedded controller for processing sensor data and controlling the actuators.
- Algorithm Development:
- Kinematics: Implement forward and inverse kinematics algorithms for calculating the position and orientation of the end-effector. Use LabVIEW’s mathematical functions for solving kinematic equations.
- Trajectory Planning: Develop a trajectory planning algorithm to generate smooth and collision-free paths. Use cubic splines and polynomial interpolation techniques.
- Force Control: Implement a force control algorithm to regulate the assembly forces. Use LabVIEW’s PID toolkit to control the force applied by the robotic arm.
- Simulation and Testing:
- Virtual Simulation: Create a virtual model of the robotic arm and simulate the assembly tasks. Validate the kinematics, trajectory planning, and force control algorithms in the simulation environment.
- Bench Testing: Test the robotic arm in a controlled environment. Verify the accuracy and repeatability of the assembly tasks.
- Deployment:
- Embedded Deployment: Deploy the application to the PXI system. Ensure precise and reliable operation in the manufacturing environment.
- Maintenance: Implement diagnostic tools for monitoring the health and performance of the robotic arm. Develop procedures for regular maintenance and software updates.
Conclusion
Developing LabVIEW applications for robotics involves a combination of graphical programming, system integration, control algorithm design, simulation, and real-world testing. LabVIEW’s intuitive interface and comprehensive libraries make it an ideal tool for creating robust and efficient robotic systems. By following the guidelines and best practices outlined in this article, you can successfully develop and deploy LabVIEW applications for a wide range of robotic applications.
Whether you are working on autonomous mobile robots, robotic arms, or complex industrial automation systems, LabVIEW provides the tools and capabilities to bring your robotic projects to life. Embrace the power of LabVIEW and explore the exciting world of robotics development!