How to Declare Variables in JavaScript
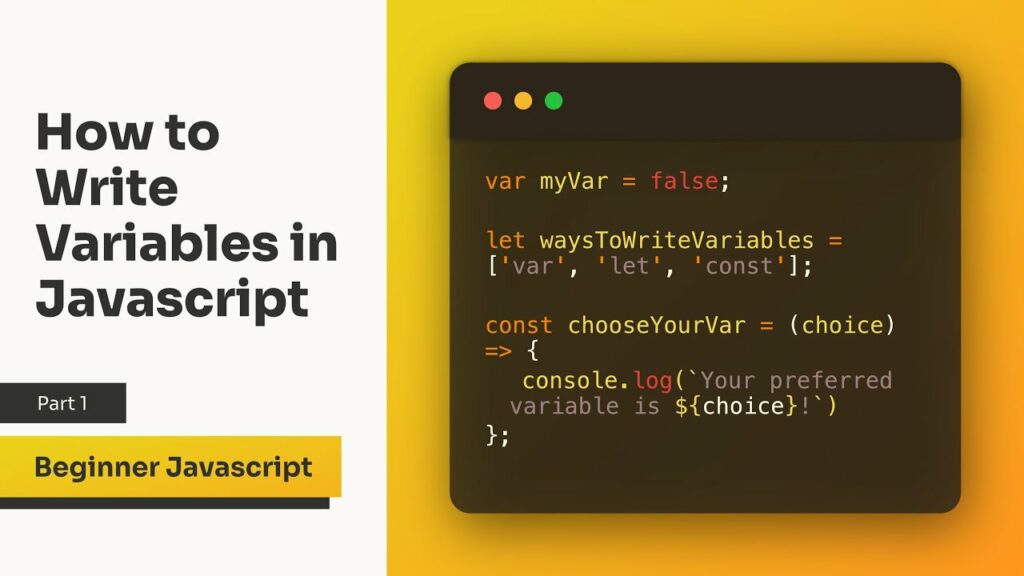
JavaScript is a versatile and powerful language used widely in web development. One of the fundamental concepts in JavaScript is variable declaration. Variables are containers for storing data values, and they play a crucial role in programming as they allow developers to create dynamic and interactive web applications. In this comprehensive guide, we will explore how to declare variables in JavaScript, including the various ways to declare them, the scope of variables, and best practices for using them effectively.
Table of Contents
- Introduction to Variables in JavaScript
- Declaring Variables with
var
- Declaring Variables with
let
- Declaring Variables with
const
- Understanding Variable Scope
- Global Scope
- Function Scope
- Block Scope
- Variable Hoisting in JavaScript
- Best Practices for Declaring Variables
- Common Mistakes and How to Avoid Them
- Conclusion
Introduction to Variables in JavaScript
Variables are named storage locations that hold data values. In JavaScript, variables can store various types of data, including numbers, strings, arrays, objects, and functions. The value of a variable can change during the execution of a program, allowing for dynamic and flexible coding.
To declare a variable in JavaScript, you use one of the following keywords: var
, let
, or const
. Each of these keywords has different characteristics and behaviors, which we will explore in detail.
Declaring Variables with var
The var
keyword is the traditional way to declare variables in JavaScript. It has been part of the language since its inception. However, it comes with some quirks that can lead to unexpected behavior if not used carefully.
Syntax
var variableName = value;
Example
var message = "Hello, world!";
var count = 42;
var isActive = true;
Characteristics of var
- Function Scope: Variables declared with
var
are function-scoped, meaning they are accessible throughout the function in which they are declared. - Hoisting: Variables declared with
var
are hoisted to the top of their scope, meaning they can be used before they are declared. However, their value will beundefined
until the declaration is encountered. - Re-declaration: Variables declared with
var
can be re-declared within the same scope without causing an error.
Function Scope Example
function exampleFunction() {
var x = 10;
if (true) {
var x = 20;
console.log(x); // Output: 20
}
console.log(x); // Output: 20
}
exampleFunction();
In this example, the variable x
declared inside the if
block overwrites the x
declared in the function scope, demonstrating the function scope of var
.
Hoisting Example
console.log(y); // Output: undefined
var y = 5;
console.log(y); // Output: 5
Here, the declaration of y
is hoisted to the top of the scope, but the assignment happens at the original line, leading to undefined
being logged first.
Re-declaration Example
var a = 1;
var a = 2;
console.log(a); // Output: 2
The re-declaration of a
does not cause an error, and the latest value is used.
Declaring Variables with let
The let
keyword was introduced in ECMAScript 6 (ES6) to address some of the limitations and issues associated with var
. It provides block scope, which is more intuitive and less error-prone.
Syntax
let variableName = value;
Example
let greeting = "Hi there!";
let age = 30;
let isLoggedIn = false;
Characteristics of let
- Block Scope: Variables declared with
let
are block-scoped, meaning they are only accessible within the block in which they are declared. - No Hoisting of Initializations: Although
let
variables are hoisted, they are not initialized until their declaration is encountered. This prevents the use oflet
variables before they are declared. - No Re-declaration: Variables declared with
let
cannot be re-declared within the same scope.
Block Scope Example
function testLet() {
let x = 10;
if (true) {
let x = 20;
console.log(x); // Output: 20
}
console.log(x); // Output: 10
}
testLet();
In this example, the variable x
declared inside the if
block is separate from the x
declared in the function scope, demonstrating the block scope of let
.
No Hoisting of Initializations Example
console.log(b); // ReferenceError: b is not defined
let b = 5;
console.log(b); // Output: 5
Here, using b
before its declaration results in a ReferenceError
because let
does not allow access to the variable before its declaration.
No Re-declaration Example
let c = 1;
let c = 2; // SyntaxError: Identifier 'c' has already been declared
Re-declaring c
within the same scope results in a syntax error.
Declaring Variables with const
The const
keyword, also introduced in ES6, is used to declare variables that should not be reassigned. It is similar to let
in terms of block scope but ensures that the variable binding remains constant.
Syntax
const variableName = value;
Example
const pi = 3.14159;
const username = "JohnDoe";
const isAdmin = true;
Characteristics of const
- Block Scope: Like
let
, variables declared withconst
are block-scoped. - Immutable Binding: The binding of a
const
variable cannot be changed once it is assigned. However, if the value is an object, the object’s properties can still be modified. - Initialization Required: A
const
variable must be initialized at the time of declaration.
Block Scope Example
function testConst() {
const x = 10;
if (true) {
const x = 20;
console.log(x); // Output: 20
}
console.log(x); // Output: 10
}
testConst();
Similar to let
, const
also respects block scope.
Immutable Binding Example
const d = 1;
d = 2; // TypeError: Assignment to constant variable.
Attempting to reassign d
results in a type error.
Initialization Required Example
const e; // SyntaxError: Missing initializer in const declaration
e = 5;
Failing to initialize e
at the time of declaration results in a syntax error.
Mutable Object Example
const user = { name: "John", age: 30 };
user.age = 31; // This is allowed
console.log(user); // Output: { name: "John", age: 31 }
While the binding of user
cannot change, the properties of the object it refers to can be modified.
Understanding Variable Scope
Variable scope determines the accessibility of variables in different parts of the code. JavaScript has three types of scope: global scope, function scope, and block scope.
Global Scope
Variables declared outside of any function or block are in the global scope. They can be accessed from anywhere in the code.
var globalVar = "I am a global variable";function accessGlobalVar() {
console.log(globalVar); // Output: I am a global variable
}
accessGlobalVar();
console.log(globalVar); // Output: I am a global variable
Function Scope
Variables declared within a function are in the function scope. They can only be accessed within that function.
function functionScopeExample() {
var localVar = "I am a local variable";
console.log(localVar); // Output: I am a local variable
}functionScopeExample();
console.log(localVar); // ReferenceError: localVar is not defined
Block Scope
Variables declared with let
or const
inside a block (e.g., within {}
) are in the block scope. They can only be accessed within that block.
if (true) {
let blockVar = "I am a block-scoped variable";
console.log(blockVar); // Output: I am a block-scoped variable
}console.log(blockVar); // ReferenceError: blockVar is not defined
Variable Hoisting in JavaScript
Hoisting is a JavaScript mechanism where variable and function declarations are moved to the top of their containing scope during the compilation phase. This means that you can use variables and functions before they are declared.
Hoisting with var
As discussed earlier, variables declared with var
are hoisted to the top of their scope but initialized with undefined
.
console.log(hoistedVar); // Output: undefined
var hoistedVar = "I am hoisted";
console.log(hoistedVar); // Output: I am hoisted
Hoisting with let
and const
Variables declared with let
and const
are also hoisted but are not initialized. Accessing them before declaration results in a ReferenceError
.
console.log(hoistedLet); // ReferenceError: Cannot access 'hoistedLet' before initialization
let hoistedLet = "I am hoisted with let";console.log(hoistedConst); // ReferenceError: Cannot access 'hoistedConst' before initialization
const hoistedConst = "I am hoisted with const";
Best Practices for Declaring Variables
To write clean, maintainable, and error-free JavaScript code, follow these best practices for declaring variables:
- Use
let
andconst
Instead ofvar
:let
andconst
provide block scope and prevent hoisting issues, making your code more predictable. - Prefer
const
for Constants: Useconst
for variables that should not be reassigned to ensure the immutability of their bindings. - Initialize Variables When Declaring: Always initialize variables when declaring them to avoid unexpected
undefined
values. - Use Meaningful Variable Names: Choose descriptive and meaningful names for your variables to make your code more readable and self-documenting.
- Avoid Global Variables: Minimize the use of global variables to prevent naming conflicts and unintended side effects.
- Use CamelCase for Variable Names: Follow the camelCase convention for variable names to maintain consistency and readability.
Common Mistakes and How to Avoid Them
Re-declaring Variables with var
Re-declaring variables with var
can lead to unexpected behavior and bugs. Use let
or const
to prevent re-declaration.
var x = 1;
var x = 2; // No error, but can cause bugs
Using Variables Before Declaration
Avoid using variables before they are declared to prevent hoisting-related issues.
console.log(a); // Output: undefined
var a = 1;
Use let
or const
to avoid this issue:
console.log(b); // ReferenceError
let b = 2;
Forgetting to Initialize const
Variables
Always initialize const
variables at the time of declaration to avoid syntax errors.
const c; // SyntaxError: Missing initializer in const declaration
c = 3;
Modifying const
Variables
Remember that const
ensures the immutability of the binding, not the value. Avoid trying to reassign const
variables.
const d = 4;
d = 5; // TypeError: Assignment to constant variable.
Using Global Variables Unnecessarily
Global variables can lead to naming conflicts and hard-to-debug issues. Use local scope as much as possible.
var globalCount = 0; // Avoid this
function increment() {
globalCount++;
}
Instead, use function or block scope:
function increment() {
let localCount = 0;
localCount++;
}
Conclusion
Declaring variables in JavaScript is a fundamental aspect of programming in the language. Understanding the differences between var
, let
, and const
, as well as their respective scopes and behaviors, is crucial for writing efficient and bug-free code. By following best practices and avoiding common mistakes, you can ensure that your JavaScript code is maintainable, predictable, and easy to debug.
Whether you are a beginner or an experienced developer, mastering variable declaration will significantly enhance your ability to build dynamic and robust JavaScript applications. Keep experimenting, stay curious, and continue to deepen your understanding of JavaScript and its nuances.