How to Generate Random Numbers and Random Data in MATLAB
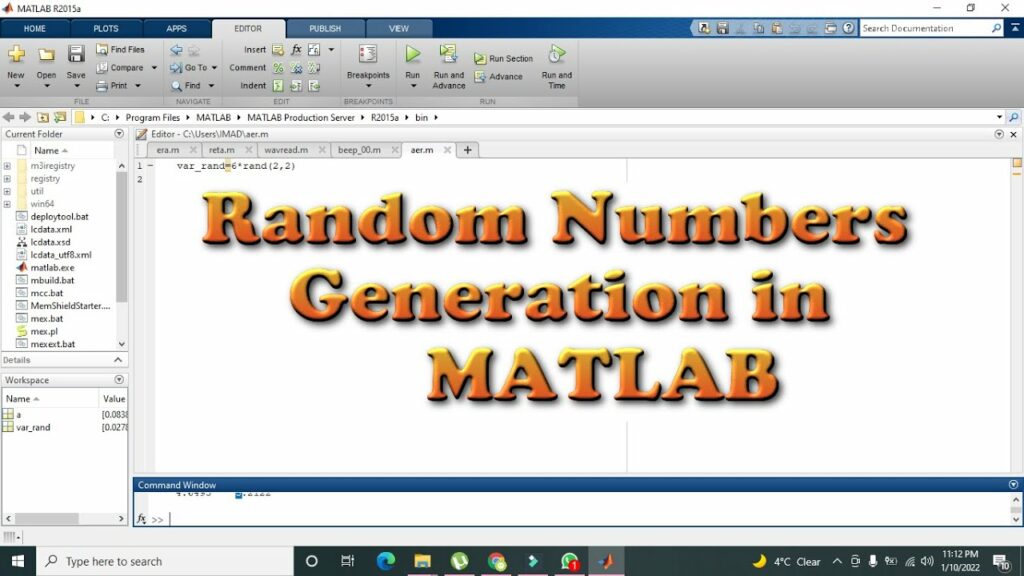
Random number generation and random data creation are crucial for various applications in scientific research, engineering, finance, and computer simulations. MATLAB offers a comprehensive set of tools for generating random numbers and random data. This article provides an extensive guide on how to generate random numbers and random data in MATLAB, covering basic and advanced methods, practical applications, and optimization tips.
Table of Contents
- Introduction to Random Number Generation in MATLAB
- Basic Random Number Generators
rand
randn
randi
randperm
- Random Number Generation from Specific Distributions
- Uniform Distribution
- Normal (Gaussian) Distribution
- Binomial Distribution
- Poisson Distribution
- Exponential Distribution
- Other Distributions
- Advanced Random Number Generation
- Controlling Random Number Generation
- Setting the Seed
- Creating Reproducible Results
- Random Data Generation
- Random Vectors and Matrices
- Random Binary Data
- Random Complex Numbers
- Random Strings and Characters
- Practical Applications
- Monte Carlo Simulations
- Random Sampling
- Stochastic Processes
- Cryptography and Security
- Optimization and Performance Tips
- Conclusion
1. Introduction to Random Number Generation in MATLAB
MATLAB provides a variety of functions to generate random numbers and data, allowing users to simulate real-world scenarios, perform statistical analysis, and develop algorithms. The built-in functions cover a wide range of distributions and can be customized to fit specific needs.
2. Basic Random Number Generators
rand
The rand
function generates uniformly distributed random numbers between 0 and 1. It can produce scalars, vectors, or matrices.
% Generate a single random number
a = rand;% Generate a 1x5 vector of random numbers
b = rand(1, 5);
% Generate a 3x3 matrix of random numbers
c = rand(3, 3);
randn
The randn
function generates random numbers from a standard normal (Gaussian) distribution with mean 0 and standard deviation 1.
% Generate a single random number from a normal distribution
d = randn;% Generate a 1x5 vector of random numbers from a normal distribution
e = randn(1, 5);
% Generate a 3x3 matrix of random numbers from a normal distribution
f = randn(3, 3);
randi
The randi
function generates uniformly distributed random integers within a specified range.
% Generate a single random integer between 1 and 10
g = randi([1, 10]);% Generate a 1x5 vector of random integers between 1 and 100
h = randi([1, 100], 1, 5);
% Generate a 3x3 matrix of random integers between -10 and 10
i = randi([-10, 10], 3, 3);
randperm
The randperm
function generates a random permutation of integers.
% Generate a random permutation of integers from 1 to 10
j = randperm(10);% Generate the first 5 integers of a random permutation from 1 to 10
k = randperm(10, 5);
3. Random Number Generation from Specific Distributions
Uniform Distribution
To generate random numbers from a uniform distribution within a specified range, you can scale and shift the output of rand
.
% Generate a 1x5 vector of random numbers from a uniform distribution between -5 and 5
l = -5 + (5 - (-5)) * rand(1, 5);
Normal (Gaussian) Distribution
To generate random numbers from a normal distribution with a specified mean and standard deviation, you can scale and shift the output of randn
.
mu = 5; % Mean
sigma = 2; % Standard deviation% Generate a 1x5 vector of random numbers from a normal distribution
m = mu + sigma * randn(1, 5);
Binomial Distribution
The binornd
function generates random numbers from a binomial distribution.
n = 10; % Number of trials
p = 0.5; % Probability of success% Generate a 1x5 vector of random numbers from a binomial distribution
n_binom = binornd(n, p, 1, 5);
Poisson Distribution
The poissrnd
function generates random numbers from a Poisson distribution.
lambda = 4; % Rate parameter% Generate a 1x5 vector of random numbers from a Poisson distribution
n_poisson = poissrnd(lambda, 1, 5);
Exponential Distribution
The exprnd
function generates random numbers from an exponential distribution.
mu = 2; % Mean% Generate a 1x5 vector of random numbers from an exponential distribution
n_expon = exprnd(mu, 1, 5);
Other Distributions
MATLAB provides functions for generating random numbers from various other distributions such as the chi-square, gamma, beta, and Weibull distributions.
% Generate a 1x5 vector of random numbers from a chi-square distribution with 2 degrees of freedom
n_chi2 = chi2rnd(2, 1, 5);% Generate a 1x5 vector of random numbers from a gamma distribution with shape parameter 2 and scale parameter 1
n_gamma = gamrnd(2, 1, 1, 5);
% Generate a 1x5 vector of random numbers from a beta distribution with parameters 2 and 5
n_beta = betarnd(2, 5, 1, 5);
% Generate a 1x5 vector of random numbers from a Weibull distribution with shape parameter 2 and scale parameter 1
n_weibull = wblrnd(2, 1, 1, 5);
4. Advanced Random Number Generation
Controlling Random Number Generation
MATLAB’s random number generation can be controlled using the rng
function, which manages the state of the random number generator.
% Save the current state of the random number generator
s = rng;% Generate random numbers
n1 = rand(1, 5);
% Restore the state of the random number generator
rng(s);
% Generate the same random numbers again
n2 = rand(1, 5);
Setting the Seed
Setting the seed for the random number generator ensures reproducibility of results. The same seed will produce the same sequence of random numbers.
% Set the seed
rng(0);% Generate random numbers
n_seeded = rand(1, 5);
Creating Reproducible Results
For reproducible results across different MATLAB sessions, you can specify the random number generator type and seed.
% Set the random number generator type and seed
rng(1, 'twister');% Generate random numbers
n_reproducible = rand(1, 5);
5. Random Data Generation
Random Vectors and Matrices
You can generate random vectors and matrices using the basic random number generation functions.
% Generate a 1x5 vector of random numbers
vector_random = rand(1, 5);% Generate a 3x3 matrix of random numbers
matrix_random = rand(3, 3);
Random Binary Data
To generate random binary data, use the randi
function with a range of [0, 1].
% Generate a 1x5 vector of random binary numbers
binary_random = randi([0, 1], 1, 5);
Random Complex Numbers
You can generate random complex numbers by combining real and imaginary parts generated using rand
or randn
.
% Generate a 1x5 vector of random complex numbers
complex_random = rand(1, 5) + 1i * rand(1, 5);
Random Strings and Characters
To generate random strings and characters, use the randi
function with appropriate ASCII ranges.
% Generate a random string of length 10
str_length = 10;
chars = ['a':'z' 'A':'Z' '0':'9']; % Characters to choose from
random_str = chars(randi(numel(chars), 1, str_length));% Generate a random character
random_char = chars(randi(numel(chars)));
6. Practical Applications
Monte Carlo Simulations
Monte Carlo simulations use random sampling to estimate complex mathematical or physical systems.
% Monte Carlo simulation to estimate the value of pi
num_samples = 1e6;
x = rand(num_samples, 1);
y = rand(num_samples, 1);
inside_circle = (x.^2 + y.^2) <= 1;
pi_estimate = 4 * sum(inside_circle) / num_samples;
disp(['Estimated value of pi: ', num2str(pi_estimate)]);
Random Sampling
Random sampling is used to select a subset of data from a larger dataset.
% Generate a dataset
dataset = 1:100;% Randomly sample 10 elements from the dataset
sampled_data = datasample(dataset, 10);
Stochastic Processes
Stochastic processes model systems that evolve over time with inherent randomness.
% Simulate a random walk
num_steps = 1000;
steps = randi([-1, 1], 1, num_steps);
position = cumsum(steps);% Plot the random walk
plot(position);
xlabel('Step');
ylabel('Position');
title('Random Walk Simulation');
Cryptography and Security
Random numbers are crucial for cryptographic algorithms and security protocols.
% Generate a random key for encryption
key_length = 16; % 128-bit key
random_key = randi([0, 255], 1, key_length, 'uint8');
7. Optimization and Performance Tips
- Preallocate Arrays: Preallocate memory for arrays to improve performance.matlab
N = 1e6;
data = zeros(1, N);
for i = 1:N
data(i) = rand;
end
- Vectorize Operations: Use vectorized operations instead of loops for faster execution.matlab
N = 1e6;
data = rand(1, N);
- Use Built-in Functions: Utilize MATLAB’s built-in functions, which are optimized for performance.matlab
% Use 'rand' instead of manually implementing random number generation
data = rand(1, 1000);
- Sparse Matrices: For large datasets with many zero elements, use sparse matrices to save memory and computation time.matlab
sparse_matrix = sparse(1000, 1000);
- Parallel Computing: For large-scale simulations or data generation, use MATLAB’s parallel computing tools.matlab
parpool; % Start parallel pool
parfor i = 1:1000
data(i) = rand;
end
delete(gcp); % Close parallel pool
8. Conclusion
Generating random numbers and random data is a fundamental task in many scientific, engineering, and computational applications. MATLAB provides a powerful and versatile set of tools for random number generation and random data creation, covering a wide range of distributions and customizations. By understanding and utilizing these tools effectively, users can perform complex simulations, statistical analysis, and algorithm development with ease and efficiency. Whether for Monte Carlo simulations, random sampling, or cryptographic applications, MATLAB’s capabilities make it an invaluable resource for generating and managing random data.