How to Use Python for Natural Language Processing with NLTK
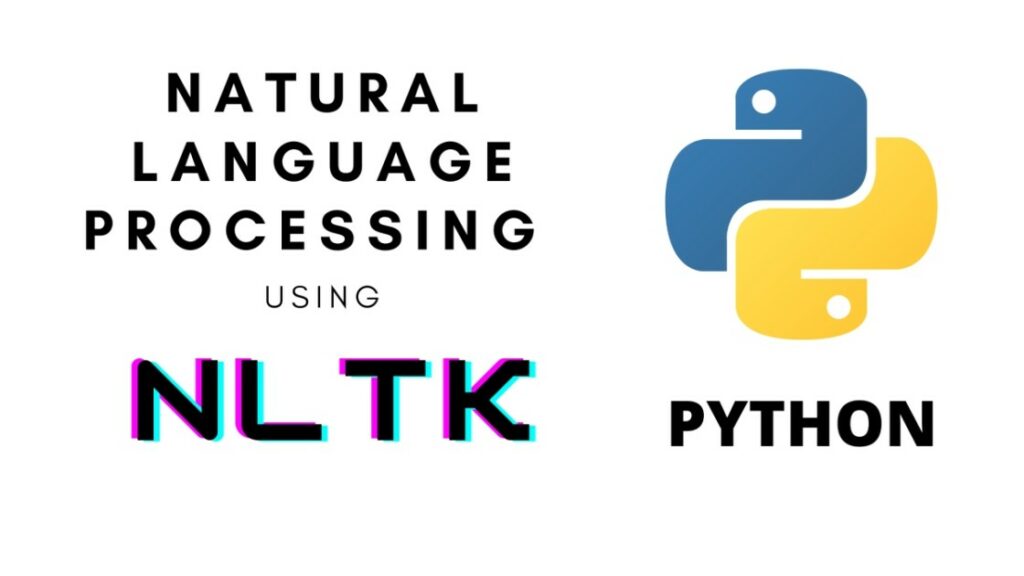
Introduction to Natural Language Processing (NLP)
Natural Language Processing (NLP) is a branch of artificial intelligence that focuses on the interaction between computers and human language. It involves tasks like understanding, interpreting, and generating human language. NLP has applications in various fields, including sentiment analysis, machine translation, text summarization, chatbots, and information retrieval.
Understanding NLTK
NLTK (Natural Language Toolkit) is a leading Python library for working with human language data. It provides a rich set of tools and resources for various NLP tasks. This comprehensive guide will explore the core concepts and functionalities of NLTK, along with practical examples to solidify your understanding.
Setting Up Your Environment
Before diving into NLP, ensure you have the necessary environment set up:
- Install Python: Download and install the latest version of Python from https://www.python.org/.
- Install NLTK: Open your terminal or command prompt and run:Bash
pip install nltk
- Download NLTK Data: Import NLTK and download necessary corpora:Python
import nltk nltk.download()
Text Preprocessing
Text preprocessing is a crucial step in NLP to prepare text data for analysis. It involves tasks like tokenization, stop word removal, stemming, and lemmatization.
Tokenization
Tokenization is the process of breaking down text into individual words or tokens.
from nltk.tokenize import word_tokenize
text = "This is a sample sentence for tokenization."
tokens = word_tokenize(text)
print(tokens)
Stop Word Removal
Stop words are common words (like “the,” “and,” “is”) that often carry little semantic meaning. Removing them can improve the efficiency and accuracy of NLP models.
from nltk.corpus import stopwords
stop_words = set(stopwords.words('english'))
filtered_tokens = [word for word in tokens if word not in stop_words]
print(filtered_tokens)
Stemming
Stemming reduces words to their root form. It’s a simpler approach but can sometimescreate words that don’t have a dictionary entry.
from nltk.stem import PorterStemmer
stemmer = PorterStemmer()
stemmed_tokens = [stemmer.stem(word) for word in filtered_tokens]
print(stemmed_tokens)
Lemmatization
Lemmatization is similar to stemming but finds the root word based on the word’s lemma, which is the dictionary form of a word. It produces more accurate results than stemming.
from nltk.stem import WordNetLemmatizer
lemmatizer = WordNetLemmatizer()
lemmatized_tokens = [lemmatizer.lemmatize(word) for word in filtered_tokens]
print(lemmatized_tokens)
Part-of-Speech Tagging
Part-of-speech (POS) tagging assigns a grammatical tag to each word in a sentence, such as noun, verb, adjective, etc.
from nltk import pos_tag
tagged_tokens = pos_tag(tokens)
print(tagged_tokens)
Named Entity Recognition (NER)
NER identifies named entities in text, such as person names, organizations, locations, dates, etc.
from nltk import ne_chunk
named_entities = ne_chunk(tagged_tokens)
print(named_entities)
Sentiment Analysis
Sentiment analysis determines the sentiment expressed in a text, whether it’s positive, negative, or neutral.
from nltk.sentiment import SentimentIntensityAnalyzer
analyzer = SentimentIntensityAnalyzer()
sentiment_scores = analyzer.polarity_scores(text)
print(sentiment_scores)
Text Classification
Text classification categorizes text into predefined classes or labels.
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.naive_bayes import MultinomialNB
# Assuming you have a dataset with text and corresponding labels
# Vectorize text data
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(text_data)
# Create and train a classifier
classifier = MultinomialNB()
classifier.fit(X, labels)
# Make predictions
new_text = ["This is a new text to classify."]
new_text_vectorized = vectorizer.transform(new_text)
predicted_label = classifier.predict(new_text_vectorized)
print(predicted_label)
Advanced NLP Topics
- Language Models: Learn about n-grams, Markov models, and recurrent neural networks for language modeling.
- Machine Translation: Explore techniques for translating text between languages.
- Text Summarization: Discover methods for generating concise summaries of lengthy text.
- Question Answering: Build systems that can answer questions based on given text.
- Dialogue Systems: Develop chatbots and virtual assistants.
Conclusion
NLTK provides a solid foundation for exploring the world of natural language processing. By mastering the concepts and techniques presented in this guide, you’ll be well-equipped to tackle various NLP challenges and build sophisticated applications.