How to Use SQLAlchemy with Python
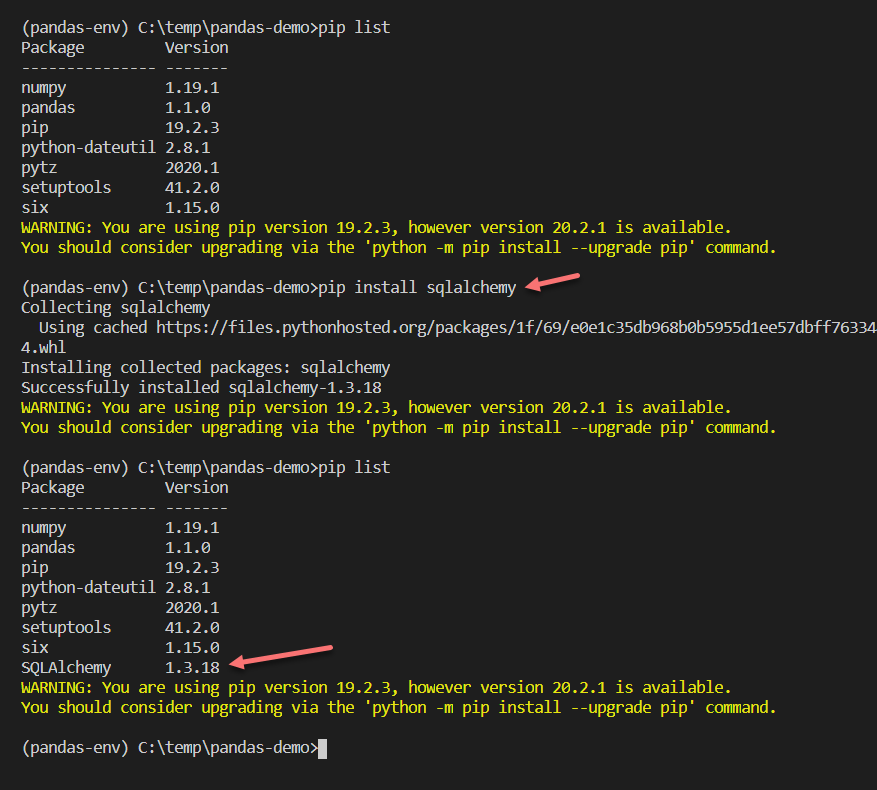
Introduction
SQLAlchemy, a powerful Python library, serves as a bridge between your Python applications and relational databases. It provides a robust and flexible framework for interacting with databases, offering features for both Object-Relational Mapping (ORM) and direct SQL execution. This comprehensive guide will delve into the intricacies of SQLAlchemy, covering everything from basic setup to advanced techniques.
Understanding SQLAlchemy’s Core Components
Before diving into practical examples, it’s essential to grasp SQLAlchemy’s fundamental building blocks:
Engine
The engine is the cornerstone of SQLAlchemy, representing the connection to your database. It encapsulates database-specific dialects and connection pooling mechanisms.
from sqlalchemy import create_engine
engine = create_engine('postgresql://user:password@host:port/database')
Session
A session is a transactional unit of work. It manages changes to objects and provides methods for querying and modifying data.
from sqlalchemy.orm import sessionmaker
Session = sessionmaker(bind=engine)
session = Session()
Declarative Base
The declarative base is a class that serves as the base for defining mapped classes. It automatically generates tables in the database based on the class definitions.
from sqlalchemy.ext.declarative import declarative_base
Base = declarative_base()
Mapped Classes
Mapped classes represent database tables as Python classes. They define columns and relationships between tables.
from sqlalchemy import Column, Integer, String
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
email = Column(String)
Object-Relational Mapping (ORM)
SQLAlchemy’s ORM layer simplifies database interactions by mapping Python objects to database tables.
Creating Tables
To create tables based on your mapped classes, use the create_all
function:
Base.metadata.create_all(engine)
Inserting Data
Create instances of your mapped classes and add them to the session:
user = User(name='John Doe', email='[email protected]')
session.add(user)
session.commit()
Querying Data
Use the session.query
method to construct queries:
users = session.query(User).all()
for user in users:
print(user.name, user.email)
Updating Data
Modify object attributes and commit the session:
user = session.query(User).filter_by(id=1).first()
user.name = 'Jane Doe'
session.commit()
Deleting Data
Delete objects from the session:
session.delete(user)
session.commit()
Advanced ORM Features
SQLAlchemy offers a rich set of features for complex data modeling and querying:
- Relationships: Define relationships between classes using
relationship
andbackref
. - Inheritance: Model class hierarchies using single table, joined table, and concrete table inheritance.
- Hybrid Properties: Combine column-based and hybrid attributes for flexible object representation.
- Querying: Leverage powerful query building capabilities with filters, joins, group by, having, and order by.
- Transactions: Manage database transactions using
session.begin()
andsession.commit()
.
Raw SQL Execution
While SQLAlchemy’s ORM is powerful, there are situations where direct SQL execution is necessary. SQLAlchemy provides the text
function for this purpose:
from sqlalchemy import text
result = session.execute(text('SELECT * FROM users WHERE name LIKE :name'), {'name': '%Doe%'}).fetchall()
Performance Optimization
SQLAlchemy offers various techniques to optimize database performance:
- Connection Pooling: Configure the engine with appropriate connection pool parameters.
- Query Optimization: Use query hints, indexes, and caching to improve query performance.
- ORM Performance: Consider using eager loading or lazy loading strategies for relationships.
- Bulk Operations: Use bulk insert, update, and delete operations for large datasets.
Error Handling and Exception Management
Proper error handling is crucial for robust applications:
- Use try-except blocks to catch exceptions.
- Handle database-specific errors gracefully.
- Log errors for debugging and monitoring.
Additional Topics
This guide has covered the essentials of SQLAlchemy. To explore further, consider the following topics:
- Database Migrations: Use tools like Alembic to manage database schema changes.
- Advanced Querying: Dive into SQLAlchemy’s query language for complex data manipulation.
- Testing: Write unit tests for your SQLAlchemy code.
- Deployment: Integrate SQLAlchemy into production environments.
- Best Practices: Follow recommended practices for efficient and maintainable SQLAlchemy code.
Conclusion
SQLAlchemy is a versatile and powerful tool for working with databases in Python. By mastering its core concepts and advanced features, you can build efficient and scalable applications. This comprehensive guide has provided a solid foundation for your SQLAlchemy journey.