How to Use the AutoCAD Electrical API
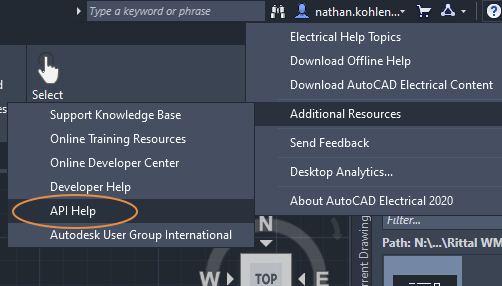
AutoCAD Electrical, part of the Autodesk suite of design tools, is a specialized software application used by electrical engineers to design electrical control systems. Beyond its extensive built-in functionalities, AutoCAD Electrical offers an API (Application Programming Interface) that allows developers to extend and customize the software according to specific needs. This comprehensive guide will cover the essentials of using the AutoCAD Electrical API, providing detailed instructions and examples to help you harness the power of this tool.
Table of Contents
- Introduction to AutoCAD Electrical API
- 1.1 Overview of the API
- 1.2 Benefits of Using the API
- 1.3 Prerequisites
- Getting Started with the AutoCAD Electrical API
- 2.1 Setting Up Your Development Environment
- 2.2 Understanding the API Documentation
- 2.3 Basic Concepts and Terminology
- Programming Languages and Tools
- 3.1 Supported Languages
- 3.2 Choosing the Right Development Tools
- AutoCAD Electrical API Basics
- 4.1 Connecting to the API
- 4.2 Basic Operations
- Working with Projects and Drawings
- 5.1 Creating and Managing Projects
- 5.2 Accessing and Modifying Drawings
- Handling Components and Symbols
- 6.1 Inserting Components
- 6.2 Editing and Deleting Components
- 6.3 Managing Symbols
- Wiring and Circuit Management
- 7.1 Creating and Modifying Wires
- 7.2 Managing Circuits
- Reports and Documentation
- 8.1 Generating Reports
- 8.2 Customizing Report Formats
- Advanced API Features
- 9.1 Event Handling
- 9.2 Custom Commands and User Interfaces
- Error Handling and Debugging
- 10.1 Common Errors and Solutions
- 10.2 Debugging Tips
- Best Practices and Optimization
- 11.1 Writing Efficient Code
- 11.2 Maintaining Code Quality
- Resources and Further Learning
- 12.1 Official Autodesk Resources
- 12.2 Community and Forums
- 12.3 Books and Tutorials
1. Introduction to AutoCAD Electrical API
1.1 Overview of the API
The AutoCAD Electrical API provides a set of programming interfaces that allow developers to automate tasks, customize workflows, and extend the capabilities of AutoCAD Electrical. By leveraging the API, users can create custom scripts, develop plugins, and integrate AutoCAD Electrical with other software systems.
1.2 Benefits of Using the API
- Automation: Automate repetitive tasks to save time and reduce errors.
- Customization: Tailor the software to meet specific workflow requirements.
- Integration: Connect AutoCAD Electrical with other applications and data sources.
- Extended Functionality: Add new features that are not available out-of-the-box.
1.3 Prerequisites
Before diving into the API, ensure you have:
- A working installation of AutoCAD Electrical.
- Basic knowledge of programming concepts.
- Familiarity with AutoCAD Electrical’s user interface and features.
2. Getting Started with the AutoCAD Electrical API
2.1 Setting Up Your Development Environment
To start using the AutoCAD Electrical API, you need to set up a development environment. Here are the steps to get started:
- Install Visual Studio: Visual Studio is a powerful IDE that supports various programming languages, including those supported by the AutoCAD Electrical API.
- Install AutoCAD Electrical SDK: The Software Development Kit (SDK) provides libraries, documentation, and samples to help you develop applications.
- Configure Environment Variables: Set up the necessary environment variables to ensure your development tools can locate the AutoCAD Electrical libraries.
2.2 Understanding the API Documentation
The API documentation is your primary resource for understanding the available functions, classes, and methods. It includes detailed descriptions, syntax, and usage examples. You can find the documentation within the SDK or on the Autodesk Developer Network (ADN) website.
2.3 Basic Concepts and Terminology
- Namespace: A namespace is a container that holds classes, methods, and other identifiers. It helps organize code and prevent naming conflicts.
- Class: A class is a blueprint for creating objects. It defines properties and methods that the objects will have.
- Method: A method is a function defined within a class that performs a specific action.
- Object: An instance of a class containing data and behavior as defined by the class.
3. Programming Languages and Tools
3.1 Supported Languages
The AutoCAD Electrical API primarily supports:
- VB.NET
- C#.NET
- VBA (Visual Basic for Applications)
3.2 Choosing the Right Development Tools
- Visual Studio: The recommended IDE for .NET development. It provides robust features for coding, debugging, and testing.
- VBA Editor: For those preferring to use VBA, the built-in editor in AutoCAD Electrical can be used for scripting and automation.
4. AutoCAD Electrical API Basics
4.1 Connecting to the API
To interact with AutoCAD Electrical, you first need to establish a connection to the API. Here’s a basic example in C#:
using Autodesk.AutoCAD.Runtime;
using Autodesk.AutoCAD.ApplicationServices;
using Autodesk.AutoCAD.DatabaseServices;
using Autodesk.AutoCAD.EditorInput;
using Autodesk.AutoCAD.Electrical.DatabaseServices;public class ApiExample
{
[ ]
public void HelloAutoCADElectrical()
{
Document doc = Application.DocumentManager.MdiActiveDocument;
Editor ed = doc.Editor;
ed.WriteMessage("Hello, AutoCAD Electrical!");
}
}
4.2 Basic Operations
Once connected, you can perform basic operations such as opening drawings, creating entities, and manipulating objects. Here’s how to create a new line in a drawing:
public void CreateLine()
{
Document doc = Application.DocumentManager.MdiActiveDocument;
Database db = doc.Database; using (Transaction tr = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)tr.GetObject(db.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)tr.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForWrite);
Line line = new Line(new Point3d(0, 0, 0), new Point3d(10, 10, 0));
btr.AppendEntity(line);
tr.AddNewlyCreatedDBObject(line, true);
tr.Commit();
}
}
5. Working with Projects and Drawings
5.1 Creating and Managing Projects
The API allows you to create, open, and manage projects programmatically. Here’s an example of creating a new project:
public void CreateProject(string projectName)
{
// Assuming necessary references and using directives are in place
ACADE_PROJECT project = new ACADE_PROJECT();
project.ProjectName = projectName;
project.SaveAs(projectName);
}
5.2 Accessing and Modifying Drawings
You can access and modify existing drawings within a project. Here’s an example of opening a drawing and modifying its properties:
public void ModifyDrawing(string drawingPath)
{
Document doc = Application.DocumentManager.Open(drawingPath, false);
using (DocumentLock docLock = doc.LockDocument())
{
Database db = doc.Database;
using (Transaction tr = db.TransactionManager.StartTransaction())
{
// Perform modifications here
tr.Commit();
}
}
}
6. Handling Components and Symbols
6.1 Inserting Components
Inserting components into a drawing involves creating instances of electrical components and placing them at specified locations:
public void InsertComponent(string blockName, Point3d position)
{
Document doc = Application.DocumentManager.MdiActiveDocument;
Database db = doc.Database; using (Transaction tr = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)tr.GetObject(db.BlockTableId, OpenMode.ForRead);
if (bt.Has(blockName))
{
BlockTableRecord btr = (BlockTableRecord)tr.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForWrite);
BlockReference blockRef = new BlockReference(position, bt[blockName]);
btr.AppendEntity(blockRef);
tr.AddNewlyCreatedDBObject(blockRef, true);
}
tr.Commit();
}
}
6.2 Editing and Deleting Components
Editing and deleting components is straightforward. Here’s an example of editing a component’s attributes:
public void EditComponentAttributes(ObjectId blockId, string attributeName, string newValue)
{
Document doc = Application.DocumentManager.MdiActiveDocument;
Database db = doc.Database; using (Transaction tr = db.TransactionManager.StartTransaction())
{
BlockReference blockRef = (BlockReference)tr.GetObject(blockId, OpenMode.ForWrite);
AttributeCollection attCol = blockRef.AttributeCollection;
foreach (ObjectId attId in attCol)
{
AttributeReference attRef = (AttributeReference)tr.GetObject(attId, OpenMode.ForWrite);
if (attRef.Tag == attributeName)
{
attRef.TextString = newValue;
break;
}
}
tr.Commit();
}
}
6.3 Managing Symbols
Managing symbols involves creating, editing, and organizing symbol libraries. Symbols are typically stored as block definitions in drawing files:
public void CreateSymbol(string blockName, List<Entity> entities)
{
Document doc = Application.DocumentManager.MdiActiveDocument;
Database db = doc.Database; using (Transaction tr = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)tr.GetObject(db.BlockTableId, OpenMode.ForWrite);
BlockTableRecord btr = new BlockTableRecord();
btr.Name = blockName;
bt.Add(btr);
tr.AddNewlyCreatedDBObject(btr, true);
foreach (Entity ent in entities)
{
btr.AppendEntity(ent);
tr.AddNewlyCreatedDBObject(ent, true);
}
tr.Commit();
}
}
7. Wiring and Circuit Management
7.1 Creating and Modifying Wires
Creating and modifying wires involves using the wire-specific classes and methods provided by the API:
public void CreateWire(Point3d start, Point3d end)
{
Document doc = Application.DocumentManager.MdiActiveDocument;
Database db = doc.Database; using (Transaction tr = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)tr.GetObject(db.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)tr.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForWrite);
// Assuming `Wire` is a class provided by the API for wire objects
Wire wire = new Wire(start, end);
btr.AppendEntity(wire);
tr.AddNewlyCreatedDBObject(wire, true);
tr.Commit();
}
}
7.2 Managing Circuits
Managing circuits includes creating, editing, and organizing circuit designs:
public void CreateCircuit(List<Wire> wires)
{
Document doc = Application.DocumentManager.MdiActiveDocument;
Database db = doc.Database; using (Transaction tr = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)tr.GetObject(db.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)tr.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForWrite);
foreach (Wire wire in wires)
{
btr.AppendEntity(wire);
tr.AddNewlyCreatedDBObject(wire, true);
}
tr.Commit();
}
}
8. Reports and Documentation
8.1 Generating Reports
The API allows for generating various types of reports, such as BOM (Bill of Materials), wire lists, and component lists:
public void GenerateReport(string reportType)
{
// Assuming there is a method to generate reports provided by the API
ReportGenerator generator = new ReportGenerator();
generator.Generate(reportType);
}
8.2 Customizing Report Formats
Reports can be customized to fit specific requirements. This involves modifying templates or using custom scripts to format the data:
public void CustomizeReportFormat(string reportType, string templatePath)
{
ReportGenerator generator = new ReportGenerator();
generator.SetTemplate(templatePath);
generator.Generate(reportType);
}
9. Advanced API Features
9.1 Event Handling
The API supports event handling, allowing you to execute custom code in response to specific events:
public void RegisterEventHandlers()
{
Document doc = Application.DocumentManager.MdiActiveDocument; doc.CommandWillStart += new CommandEventHandler(OnCommandWillStart);
doc.CommandEnded += new CommandEventHandler(OnCommandEnded);
}
private void OnCommandWillStart(object sender, CommandEventArgs e)
{
// Custom logic before a command starts
}
private void OnCommandEnded(object sender, CommandEventArgs e)
{
// Custom logic after a command ends
}
9.2 Custom Commands and User Interfaces
You can create custom commands and user interfaces to enhance the user experience:
[
public void CustomCommand()
{
Document doc = Application.DocumentManager.MdiActiveDocument;
Editor ed = doc.Editor; ed.WriteMessage("This is a custom command!");
}
]Custom user interfaces can be created using .NET Windows Forms or WPF:
public void ShowCustomForm()
{
CustomForm form = new CustomForm();
Application.ShowModalDialog(form);
}
10. Error Handling and Debugging
10.1 Common Errors and Solutions
Common Error: Null Reference Exception Solution: Ensure that objects are properly initialized before accessing their members.
Common Error: Invalid Cast Exception Solution: Verify that you are casting objects to the correct type.
10.2 Debugging Tips
- Use Breakpoints: Set breakpoints in your code to pause execution and inspect variables.
- Log Messages: Use logging to record messages and errors for later analysis.
- Exception Handling: Implement try-catch blocks to handle exceptions gracefully.
11. Best Practices and Optimization
11.1 Writing Efficient Code
- Optimize Loops: Minimize the use of nested loops and complex calculations within loops.
- Use Efficient Data Structures: Choose the appropriate data structures for your needs to improve performance.
- Cache Results: Cache results of expensive operations to avoid redundant calculations.
11.2 Maintaining Code Quality
- Code Reviews: Regularly review code with peers to ensure quality and adherence to best practices.
- Unit Testing: Write unit tests to validate the functionality of your code.
- Documentation: Maintain thorough documentation for your code to improve maintainability.
12. Resources and Further Learning
12.1 Official Autodesk Resources
- Autodesk Developer Network (ADN): Offers comprehensive resources, including documentation, SDKs, and forums.
- AutoCAD Electrical Help: Built-in help documentation within the software.
12.2 Community and Forums
- AutoCAD Forums: Engage with other users and developers to share knowledge and solutions.
- Stack Overflow: Ask questions and find answers related to AutoCAD Electrical API development.
12.3 Books and Tutorials
- Mastering AutoCAD Electrical: Comprehensive guides and tutorials for mastering AutoCAD Electrical and its API.
- Online Courses: Various online platforms offer courses on AutoCAD Electrical and API development.
By following this guide, you will be well-equipped to leverage the AutoCAD Electrical API to customize and extend the software to meet your specific needs. Whether you’re automating tasks, integrating with other systems, or developing new features, the API provides powerful tools to enhance your workflow and productivity.