Implementing Image Processing and Computer Vision in LabVIEW
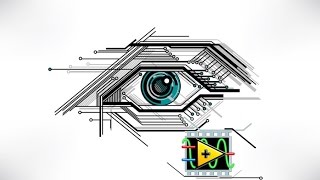
Image processing and computer vision are powerful technologies that enable machines to interpret and understand visual information from the world. These technologies are used in various fields, including industrial automation, medical imaging, robotics, and surveillance. LabVIEW, a graphical programming environment developed by National Instruments, provides a comprehensive platform for developing and implementing image processing and computer vision applications. This article delves into the intricacies of implementing these technologies in LabVIEW, covering fundamental concepts, tools, techniques, and best practices.
Introduction to Image Processing and Computer Vision
What is Image Processing?
Image processing involves manipulating and analyzing digital images to improve their quality or extract useful information. It encompasses a wide range of operations, including filtering, enhancement, segmentation, and feature extraction. The main goals of image processing are:
- Enhancement: Improving the visual appearance of images for better interpretation by humans.
- Restoration: Correcting defects and distortions in images.
- Analysis: Extracting quantitative information from images.
What is Computer Vision?
Computer vision is a field of artificial intelligence (AI) that enables computers to interpret and make decisions based on visual data. It involves the development of algorithms and models to understand and analyze the content of images and videos. The primary goals of computer vision are:
- Recognition: Identifying objects, patterns, and features in images.
- Tracking: Following the movement of objects in video sequences.
- Interpretation: Understanding the scene and context within images and videos.
Applications of Image Processing and Computer Vision
- Industrial Automation: Quality control, defect detection, and robotic guidance.
- Medical Imaging: Diagnosing diseases, image-guided surgery, and radiology.
- Surveillance: Security monitoring, motion detection, and facial recognition.
- Robotics: Navigation, object manipulation, and autonomous operation.
- Augmented Reality: Enhancing real-world environments with computer-generated information.
LabVIEW for Image Processing and Computer Vision
Overview of LabVIEW
LabVIEW (Laboratory Virtual Instrument Engineering Workbench) is a graphical programming environment widely used for data acquisition, instrument control, and industrial automation. It uses a dataflow programming model where functions are represented as nodes and data flows between them through wires. Key features of LabVIEW include:
- Graphical Programming: Intuitive block diagram approach to programming.
- Comprehensive Libraries: Extensive libraries for data acquisition, signal processing, and analysis.
- Real-Time Capabilities: Support for real-time applications and embedded systems.
- Integration: Seamless integration with hardware devices and other software platforms.
Vision Development Module
The Vision Development Module (VDM) is an add-on for LabVIEW that provides tools for developing image processing and computer vision applications. It includes a rich set of functions for acquiring, processing, analyzing, and displaying images. Key components of VDM include:
- Image Acquisition: Interfaces for acquiring images from cameras and other imaging devices.
- Image Processing: Functions for filtering, transforming, and enhancing images.
- Image Analysis: Tools for feature extraction, pattern matching, and measurement.
- Machine Vision: High-level functions for object detection, tracking, and inspection.
- Visualization: Tools for displaying and annotating images.
Developing Image Processing Applications in LabVIEW
Image Acquisition
- Camera Interfaces: LabVIEW supports various camera interfaces, including USB, GigE, FireWire, and Camera Link. Use the IMAQdx driver for acquiring images from these cameras.
- Frame Grabbers: For high-speed and high-resolution image acquisition, use frame grabbers that interface with cameras and digitize the image data.
- Acquisition Functions: Use functions like
IMAQdx Open Camera
,IMAQdx Configure Grab
, andIMAQdx Grab
to acquire images in LabVIEW.
Image Processing Techniques
- Filtering:
- Spatial Filters: Apply spatial filters like smoothing, sharpening, and edge detection to enhance image features. Use functions like
IMAQ Convolute
,IMAQ Edge Detection
, andIMAQ Smooth
. - Frequency Domain Filters: Transform images to the frequency domain using Fourier transform, apply filters, and then transform back to the spatial domain. Use functions like
IMAQ FFT
andIMAQ Inverse FFT
.
- Spatial Filters: Apply spatial filters like smoothing, sharpening, and edge detection to enhance image features. Use functions like
- Morphological Operations:
- Erosion and Dilation: Use these operations to remove noise and fill gaps in binary images. Use functions like
IMAQ Morphology
. - Opening and Closing: Combine erosion and dilation to smooth boundaries and eliminate small objects. Use functions like
IMAQ Morphology
.
- Erosion and Dilation: Use these operations to remove noise and fill gaps in binary images. Use functions like
- Thresholding and Segmentation:
- Thresholding: Convert grayscale images to binary images based on a threshold value. Use functions like
IMAQ Threshold
. - Segmentation: Divide an image into regions based on properties like intensity, color, or texture. Use functions like
IMAQ Region of Interest
.
- Thresholding: Convert grayscale images to binary images based on a threshold value. Use functions like
- Geometric Transformations:
- Translation, Rotation, and Scaling: Transform images to correct orientation, position, and size. Use functions like
IMAQ Rotate
andIMAQ Scale
. - Affine and Perspective Transforms: Apply more complex geometric transformations for tasks like image rectification. Use functions like
IMAQ Transform
.
- Translation, Rotation, and Scaling: Transform images to correct orientation, position, and size. Use functions like
Image Analysis
- Feature Extraction:
- Edge Detection: Identify edges in images using methods like Sobel, Canny, and Laplacian. Use functions like
IMAQ Edge Detection
. - Corner Detection: Detect corners and interest points in images using algorithms like Harris and Shi-Tomasi. Use functions like
IMAQ Find Corners
. - Blob Analysis: Analyze connected components in binary images to extract features like area, centroid, and perimeter. Use functions like
IMAQ Particle Analysis
.
- Edge Detection: Identify edges in images using methods like Sobel, Canny, and Laplacian. Use functions like
- Pattern Matching:
- Template Matching: Locate predefined templates in images using correlation-based methods. Use functions like
IMAQ Match Pattern
. - Geometric Matching: Find shapes and objects in images based on geometric properties. Use functions like
IMAQ Match Geometric Pattern
.
- Template Matching: Locate predefined templates in images using correlation-based methods. Use functions like
- Measurement:
- Distance and Angle: Measure distances and angles between features in images. Use functions like
IMAQ Measure Distances
. - Area and Perimeter: Calculate the area and perimeter of objects in images. Use functions like
IMAQ Measure Area
.
- Distance and Angle: Measure distances and angles between features in images. Use functions like
Machine Vision Applications
- Object Detection and Recognition:
- Use trained classifiers and neural networks to detect and recognize objects in images. Integrate with machine learning libraries for advanced applications.
- Object Tracking:
- Implement tracking algorithms to follow the movement of objects in video sequences. Use functions like
IMAQ Tracking
.
- Implement tracking algorithms to follow the movement of objects in video sequences. Use functions like
- Quality Inspection:
- Develop automated inspection systems to detect defects and ensure product quality. Use a combination of image processing and analysis techniques.
Visualization and User Interface
- Image Display:
- Use
IMAQ WindDraw
and other display functions to visualize images in LabVIEW. - Create custom user interfaces for interactive image analysis and control.
- Use
- Annotation:
- Add annotations like lines, circles, and text to images for better interpretation. Use functions like
IMAQ Overlay
.
- Add annotations like lines, circles, and text to images for better interpretation. Use functions like
- Graphical User Interface (GUI):
- Design intuitive GUIs using LabVIEW’s front panel to provide user interaction and control.
Case Studies
Case Study 1: Automated Optical Inspection (AOI) System
Project Overview: Develop an AOI system for inspecting printed circuit boards (PCBs) in an electronics manufacturing line.
- Image Acquisition:
- Use high-resolution cameras and frame grabbers to capture images of PCBs moving on a conveyor belt.
- Integrate lighting systems to ensure uniform illumination and minimize shadows.
- Image Processing:
- Apply filters to enhance the contrast and remove noise.
- Use edge detection and thresholding to segment the components and tracks on the PCB.
- Image Analysis:
- Perform template matching to identify and locate components.
- Conduct blob analysis to measure the dimensions and positions of tracks and pads.
- Use geometric matching to detect misalignments and missing components.
- Machine Vision:
- Implement defect detection algorithms to identify issues like soldering defects, missing components, and misalignments.
- Use pattern recognition to classify defects and categorize their severity.
- Visualization and Reporting:
- Display inspection results in real-time on a user interface.
- Annotate images with detected defects and measurements.
- Generate detailed inspection reports for quality control and traceability.
Case Study 2: Medical Imaging System
Project Overview: Develop a medical imaging system for analyzing ultrasound images to assist in diagnosing medical conditions.
- Image Acquisition:
- Use ultrasound transducers to capture images of internal body structures.
- Interface with ultrasound machines to acquire image data in real-time.
- Image Processing:
- Apply filters to enhance image quality and remove speckle noise.
- Use morphological operations to segment anatomical structures of interest.
- Image Analysis:
- Perform edge detection to outline structures like organs and blood vessels.
- Use pattern matching to identify specific features or abnormalities.
- Measurement and Diagnosis:
- Measure distances, areas, and volumes of anatomical structures.
- Compare measurements against standard reference values to assist in diagnosis.
- Visualization and Reporting:
- Display ultrasound images with annotated measurements and analysis results.
- Generate diagnostic reports with images and quantitative data.
Best Practices for Implementing Image Processing and Computer Vision in LabVIEW
Planning and Design
- Define Clear Objectives: Clearly outline the goals and requirements of the application, including the types of images to be processed and the desired outcomes.
- Select Appropriate Hardware: Choose cameras, frame grabbers, and lighting systems that meet the application’s requirements.
- Design for Scalability: Design the system to accommodate future expansion and upgrades.
Development and Implementation
- Modular Design: Develop modular and reusable components to simplify maintenance and updates.
- Optimization: Optimize image processing algorithms for speed and efficiency, especially for real-time applications.
- Error Handling: Implement robust error handling to detect and manage errors gracefully.
Testing and Validation
- Calibration: Regularly calibrate cameras and imaging systems to maintain accuracy.
- Validation: Rigorously test the system using a diverse set of images to ensure reliability and robustness.
- Documentation: Maintain comprehensive documentation of the system design, development process, and test procedures.
Maintenance and Support
- Regular Maintenance: Perform regular maintenance to ensure the system continues to operate reliably.
- Updates and Upgrades: Update software and hardware components to incorporate new features and improvements.
- User Training: Provide training and support to users to ensure they can effectively use the system.
Future Trends in Image Processing and Computer Vision
Deep Learning and AI
Deep learning and AI are transforming the field of image processing and computer vision. Convolutional neural networks (CNNs) and other deep learning models are achieving state-of-the-art performance in tasks like object detection, image segmentation, and recognition. LabVIEW’s integration with machine learning frameworks will enable the development of advanced AI-driven vision applications.
Edge Computing
Edge computing involves processing data at the edge of the network, closer to the source of the data. This approach reduces latency and bandwidth requirements, making it ideal for real-time image processing applications. LabVIEW’s support for embedded systems and real-time processing will facilitate the implementation of edge-based vision systems.
3D Vision
3D vision is becoming increasingly important in applications like robotics, industrial automation, and augmented reality. Techniques like stereo vision, structured light, and time-of-flight are used to capture and analyze 3D images. LabVIEW’s capabilities for handling 3D data will enable the development of sophisticated 3D vision applications.
Augmented Reality (AR)
AR technology overlays computer-generated information onto the real world, enhancing the user’s perception and interaction with their environment. Image processing and computer vision are key components of AR systems. LabVIEW’s integration with AR frameworks will support the development of AR applications for various industries.
Quantum Computing
Quantum computing has the potential to revolutionize image processing and computer vision by solving complex problems much faster than classical computers. As quantum computing technology matures, it may become an important tool for advanced vision applications. LabVIEW’s adaptability will allow it to integrate with quantum computing platforms in the future.
Conclusion
Implementing image processing and computer vision in LabVIEW involves a comprehensive process of planning, design, development, and validation. By leveraging the capabilities of LabVIEW and the Vision Development Module, developers can create powerful and efficient vision applications for a wide range of industries. Understanding the fundamental concepts, tools, and techniques, and following best practices, will ensure the successful implementation of these technologies. As technology continues to evolve, staying abreast of emerging trends and advancements in image processing and computer vision will be essential for maintaining competitiveness and driving innovation.