NumPy: The Foundation of Numerical Computing in Python
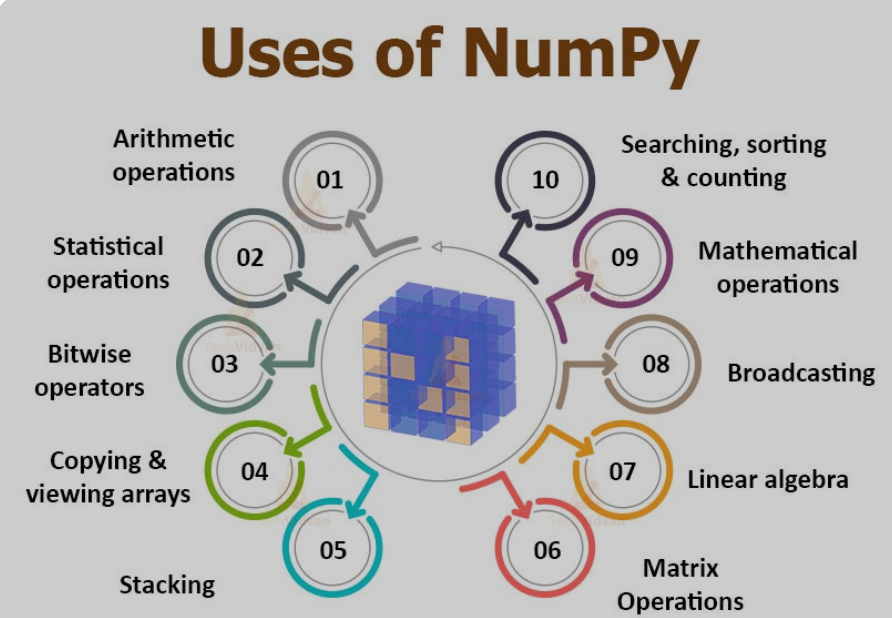
Introduction
NumPy, short for Numerical Python, is a fundamental library for scientific computing in Python. It provides high-performance multidimensional array objects, along with a vast collection of mathematical functions to operate on these arrays. NumPy is the cornerstone for many scientific Python libraries, including Pandas, SciPy, and Matplotlib. This comprehensive guide will delve into the intricacies of NumPy, covering everything from basic array creation to advanced linear algebra and Fourier transforms.
Understanding NumPy Arrays
Creating NumPy Arrays
- From Python lists:Python
import numpy as np my_list = [1, 2, 3, 4, 5] my_array = np.array(my_list)
- Using built-in functions:
np.zeros()
: Create an array of zerosnp.ones()
: Create an array of onesnp.arange()
: Create an array with evenly spaced valuesnp.linspace()
: Create an array with evenly spaced numbers over a specified intervalnp.random.rand()
: Create an array of random numbersnp.random.randn()
: Create an array of random numbers from a standard normal distribution
- From files:
np.loadtxt()
: Load data from a text filenp.genfromtxt()
: Load data from a more flexible text format
Array Attributes and Methods
shape
: Get the dimensions of the arraydtype
: Get the data type of the array elementsndim
: Get the number of dimensionssize
: Get the total number of elementsreshape()
: Reshape the arraytranspose()
: Transpose the arrayflatten()
: Flatten the array into a one-dimensional arrayravel()
: Return a contiguous flattened array
Basic Array Operations
NumPy supports element-wise arithmetic operations, comparison operators, and logical operations on arrays.
- Arithmetic operations:
+
,-
,*
,/
,//
,%
,**
- Comparison operators:
==
,!=
,<
,>
,<=
,>=
- Logical operations:
&
,|
,~
Indexing and Slicing
NumPy provides powerful indexing and slicing capabilities for accessing and manipulating array elements.
- Basic slicing:
array[start:stop:step]
- Integer indexing:
array[index_array]
- Boolean indexing:
array[boolean_mask]
Advanced Array Manipulation
- Concatenation:
np.concatenate()
,np.vstack()
,np.hstack()
- Splitting:
np.split()
,np.vsplit()
,np.hsplit()
- Stacking:
np.stack()
,np.hstack()
,np.vstack()
- Reshaping:
np.reshape()
,np.ravel()
,np.flatten()
Linear Algebra with NumPy
NumPy provides functions for linear algebra operations:
np.dot()
: Matrix multiplicationnp.linalg.inv()
: Matrix inversionnp.linalg.solve()
: Solve linear equationsnp.linalg.eig()
: Compute eigenvalues and eigenvectorsnp.linalg.svd()
: Singular value decomposition
Broadcasting
Broadcasting is a powerful feature that allows NumPy to perform operations on arrays of different shapes. NumPy automatically broadcasts the smaller array to match the shape of the larger array.
NumPy for Performance
NumPy is optimized for performance, especially when working with large datasets. Key factors for performance improvement include:
- Vectorization: Performing operations on entire arrays instead of using loops.
- Memory-efficient operations: Avoiding unnecessary memory copies.
- Leveraging NumPy’s optimized functions.
Applications of NumPy
NumPy has a wide range of applications in scientific computing, including:
- Image and signal processing
- Data analysis and machine learning
- Numerical simulations
- Financial modeling
- Scientific visualization
Conclusion
NumPy is a cornerstone library for numerical computing in Python. Its powerful array operations, linear algebra functions, and broadcasting capabilities make it an essential tool for data scientists, engineers, and researchers. This guide has provided a comprehensive overview, but there is always more to explore. Experiment with different datasets and explore advanced techniques to enhance your numerical computing skills.