How to Solve Systems of Linear Equations Using MATLAB
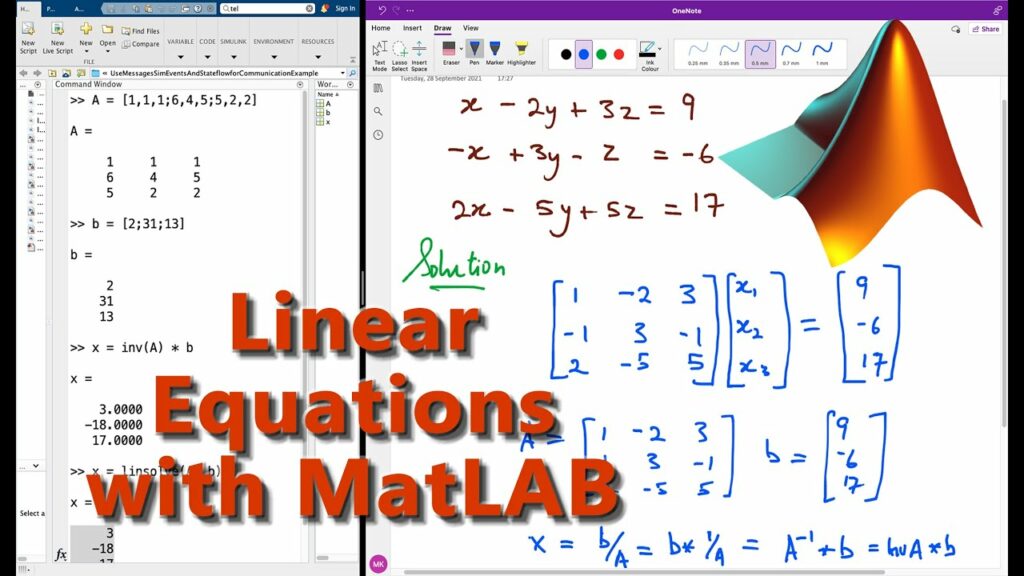
Systems of linear equations are a cornerstone in various fields such as engineering, physics, computer science, and economics. MATLAB, a high-performance language for technical computing, offers powerful tools to solve these systems efficiently. This article provides a comprehensive guide on solving systems of linear equations using MATLAB, covering various methods and practical applications.
Table of Contents
- Introduction to Systems of Linear Equations
- Basic Concepts and Definitions
- Linear Equations
- Matrices and Vectors
- Types of Solutions
- Methods for Solving Linear Systems
- Direct Methods
- Gaussian Elimination
- LU Decomposition
- Iterative Methods
- Jacobi Method
- Gauss-Seidel Method
- Conjugate Gradient Method
- Direct Methods
- Solving Linear Systems in MATLAB
- Using the Backslash Operator
- Using Matrix Inversion
- Using Decomposition Methods
- Using Iterative Methods
- Handling Special Cases
- Underdetermined Systems
- Overdetermined Systems
- Sparse Systems
- Practical Applications
- Electrical Circuits
- Structural Analysis
- Network Flows
- Data Fitting and Regression
- Optimization and Performance Tips
- Conclusion
1. Introduction to Systems of Linear Equations
A system of linear equations consists of multiple linear equations involving the same set of variables. These systems are fundamental in modeling and solving real-world problems across various scientific and engineering disciplines. MATLAB provides an extensive suite of functions to handle these systems, making it a valuable tool for both theoretical and applied work.
2. Basic Concepts and Definitions
Linear Equations
A linear equation in nn variables is an equation of the form:
a1x1+a2x2+⋯+anxn=ba_1x_1 + a_2x_2 + \cdots + a_nx_n = b
where a1,a2,…,ana_1, a_2, \ldots, a_n and bb are constants, and x1,x2,…,xnx_1, x_2, \ldots, x_n are the variables.
Matrices and Vectors
A system of linear equations can be represented in matrix form as:
Ax=bA\mathbf{x} = \mathbf{b}
where AA is an m×nm \times n matrix of coefficients, x\mathbf{x} is an n×1n \times 1 vector of variables, and b\mathbf{b} is an m×1m \times 1 vector of constants.
Types of Solutions
A system of linear equations can have:
- A unique solution
- Infinitely many solutions
- No solution
The nature of the solution depends on the properties of the matrix AA, such as its rank and determinant.
3. Methods for Solving Linear Systems
Direct Methods
Gaussian Elimination
Gaussian elimination is a systematic method for solving systems of linear equations. It involves transforming the matrix AA into an upper triangular form using a series of row operations, followed by back substitution.
LU Decomposition
LU decomposition factors a matrix AA into a product of a lower triangular matrix LL and an upper triangular matrix UU. This method simplifies solving the system Ax=bA\mathbf{x} = \mathbf{b} by breaking it into two simpler systems:
Ly=bL\mathbf{y} = \mathbf{b} Ux=yU\mathbf{x} = \mathbf{y}
Iterative Methods
Iterative methods are used for solving large systems of linear equations, especially when direct methods are computationally expensive.
Jacobi Method
The Jacobi method is an iterative algorithm that updates each variable in the system independently based on the previous iteration’s values.
Gauss-Seidel Method
The Gauss-Seidel method improves upon the Jacobi method by using the latest updated values for the variables within each iteration.
Conjugate Gradient Method
The conjugate gradient method is an efficient iterative method for solving sparse, symmetric positive-definite systems of linear equations.
4. Solving Linear Systems in MATLAB
Using the Backslash Operator
The backslash operator (\
) is MATLAB’s most efficient and preferred method for solving linear systems. It automatically selects the best algorithm based on the properties of the matrix AA.
A = [3, 2; 1, 2];
b = [2; 0];
x = A \ b;
disp(x);
Using Matrix Inversion
Although not recommended due to numerical stability and computational efficiency concerns, matrix inversion can be used to solve linear systems.
x = inv(A) * b;
disp(x);
Using Decomposition Methods
MATLAB provides functions for LU, QR, and Cholesky decomposition, which can be used to solve linear systems.
LU Decomposition
[L, U] = lu(A);
y = L \ b;
x = U \ y;
disp(x);
QR Decomposition
[Q, R] = qr(A);
y = Q' * b;
x = R \ y;
disp(x);
Cholesky Decomposition
Applicable for symmetric positive-definite matrices.
R = chol(A);
y = R' \ b;
x = R \ y;
disp(x);
Using Iterative Methods
MATLAB provides iterative solvers such as bicg
, gmres
, and pcg
.
Conjugate Gradient Method
x = pcg(A, b);
disp(x);
5. Handling Special Cases
Underdetermined Systems
Underdetermined systems have more variables than equations. MATLAB’s lsqminnorm
function finds the minimum-norm solution.
x = lsqminnorm(A, b);
disp(x);
Overdetermined Systems
Overdetermined systems have more equations than variables. MATLAB’s lsqnonneg
function solves these systems using the least squares approach.
x = lsqnonneg(A, b);
disp(x);
Sparse Systems
For large, sparse systems, MATLAB’s sparse matrix functions and iterative solvers are highly efficient.
A = sparse([1, 2; 2, 3; 3, 1]);
b = [1; 2; 3];
x = bicg(A, b);
disp(x);
6. Practical Applications
Electrical Circuits
In electrical engineering, systems of linear equations are used to analyze circuits using Kirchhoff’s laws.
A = [1, -1, 0; -1, 2, -1; 0, -1, 1];
b = [5; 0; 5];
x = A \ b;
disp('Currents in the circuit:');
disp(x);
Structural Analysis
Structural analysis involves solving systems of linear equations to determine the forces and displacements in structures.
A = [10, -2, 0; -2, 10, -2; 0, -2, 10];
b = [100; 0; 0];
x = A \ b;
disp('Displacements in the structure:');
disp(x);
Network Flows
In operations research, linear systems are used to optimize network flows, such as transportation and logistics.
A = [1, 1, 0; 0, 1, 1; 1, 0, 1];
b = [50; 60; 70];
x = A \ b;
disp('Flow in the network:');
disp(x);
Data Fitting and Regression
Linear regression involves solving systems of linear equations to find the best-fit line for a set of data points.
X = [1, 1; 1, 2; 1, 3; 1, 4];
y = [2; 2.5; 3; 3.5];
coeff = X \ y;
disp('Regression coefficients:');
disp(coeff);
7. Optimization and Performance Tips
- Preallocation: Preallocate memory for matrices to avoid dynamically resizing them, which can be slow.matlab
A = zeros(1000, 1000);
- Vectorization: Use vectorized operations instead of loops for better performance.matlab
A = (1:1000).^2;
- Sparse Matrices: Use sparse matrices for large systems with many zero elements to save memory and computation time.matlab
S = sparse(A);
- Efficient Solvers: Use MATLAB’s efficient solvers like the backslash operator (
\
) and iterative solvers for large systems.
8. Conclusion
Solving systems of linear equations is a fundamental task in many scientific and engineering applications. MATLAB provides powerful and efficient tools for handling these systems, from basic matrix operations to advanced decomposition methods and iterative solvers. By leveraging MATLAB’s capabilities, you can solve complex linear systems accurately and efficiently, making it an invaluable tool for researchers and engineers.